Coding Proficiency
We expect you to understand the code that you wrote. It can be difficult to be sure that you understand code that is very similar to pseudocode that you read, so this question is intended to force you to approach the problem a way that differs from your original approach.
def alphabeta_eval (edges, values,
node: int,
is_max : bool,
bounds: [int]):
return 0
The inputs to your function are:
- edges: an adjacency dictionary
- values: the list of numbers, that represents a complete binary tree (just like for the code you wrote)
- node: the node number you are evaluating (root node is 0)
- is_max: true for the maximizing player, false for the minimizing player
- bounds: a list with two numbers, the lower and upper bounds for the possible values of the game (this should be started with $[-\infty,\infty]$)
Output the number of leaf nodes used. Set the values of all of the nodes that you compute by changing the values list. (Do not change the values of the nodes that get pruned.)
Walkthrough Example
In the example below, before running:
edges = {0:[1, 2], 1:[3, 4], 2:[5, 6], 3:[], 4:[], 5:[], 6:[]}
values = [0,0,0,12,-7,-17,-15]
Then running
count = alphabeta_eval(edges, values, 0, True, [-math.inf, math.inf])
should produce
count == 3
values == [-7,-7,-17,12,-7,-17,-15]
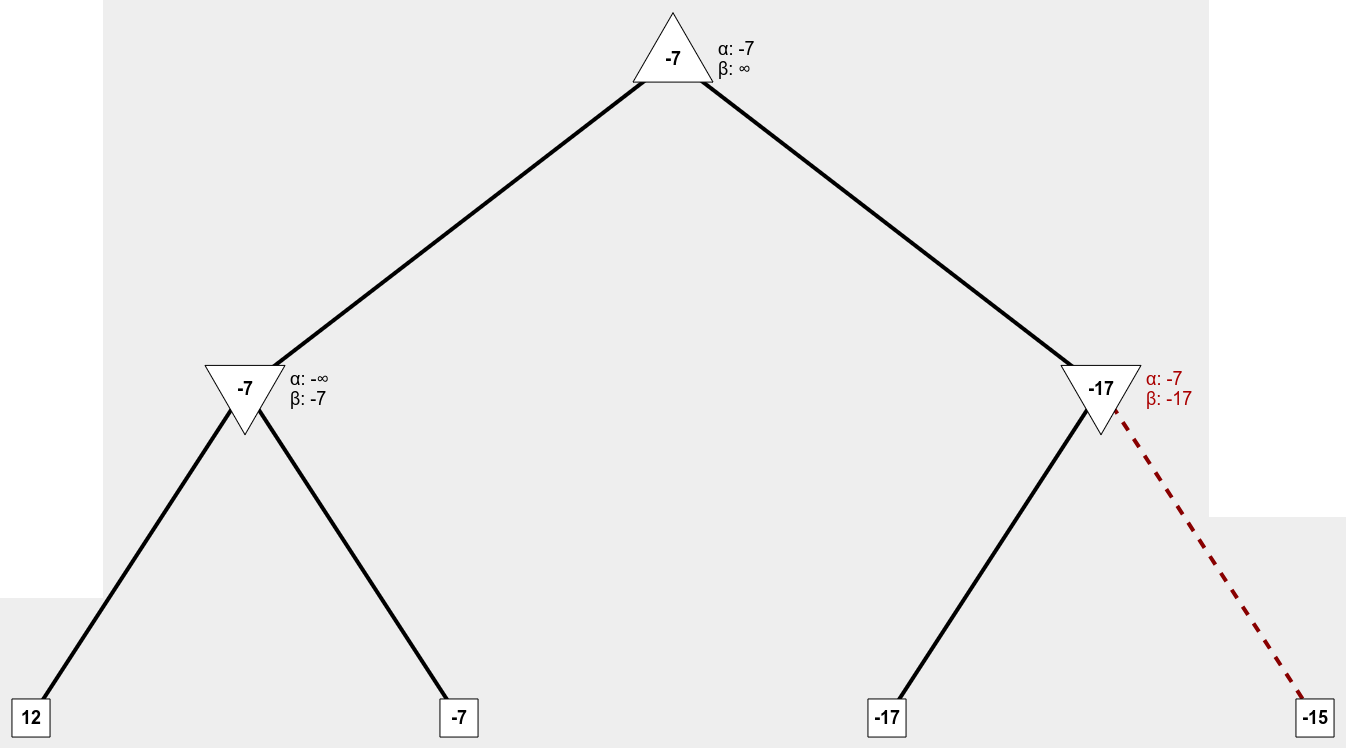
Comments
You should not immediately move all of the information into variables that have the exact same variable names as your memorized alpha-beta pseudocode. Try thinking with different variable names to expand your understanding. The closer you have to stick to memorized names, the quicker you will forget everything about how it works.