Circle Grid
In this sequence of exercises, you will create a grid of circles and then have one highlighted when you click on it.
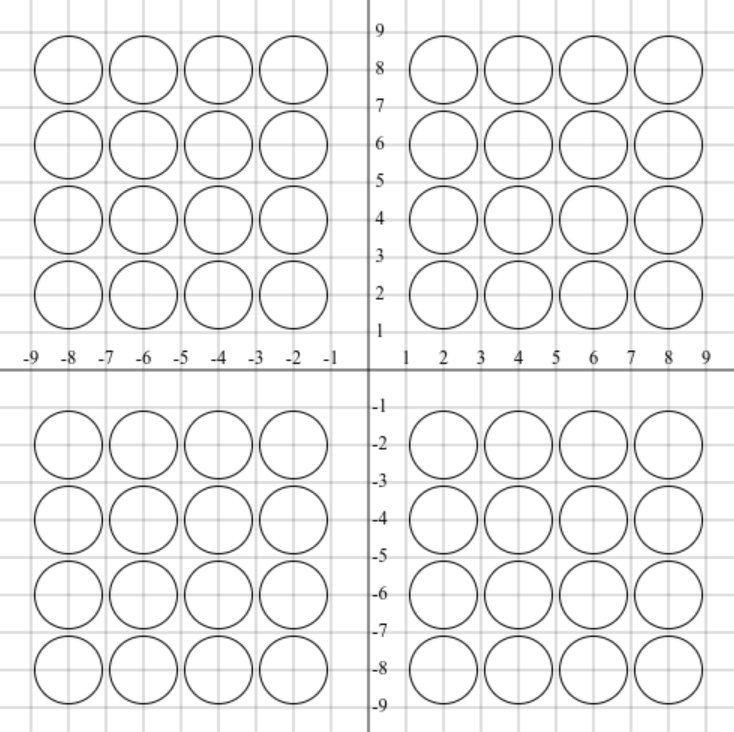
-
Each circle shown above has radius 0.9 and is centered on a grid. The centers are (2,2), (2,4), (2,6), (4,2), (4,4), etc. Make a variable containing the centers of all of the circles.
-
Make a
Picture
of the image shown above. -
When you click on the screen, the program needs to determine which circle you clicked on. As a warmup to that, write a function that takes in a list of centers and point, and puts out the center of the circle closest to the point. If there is more than one “closest” center, return any one of them.
nearest_center :: [Point] -> Point -> Point
-
Now you want actually want to determine which circle got clicked on, and ignore the click if it wasn’t in any of the circles. You could return
[Point]
and if the list is empty the click was not in any of the circles. If you know how to useMaybe
in Haskell, the return typeMaybe Point
is good. -
Make an “activity” that highlights the last circle that was clicked, using a
Point
for your model. -
Make an activity that highlights all of the circles that get clicked, using
[Point]
for your model. -
Modify your activity so that clicking on a highlighted circle removes the highlighting.
-
You now have something quite close to the game of Lights Out. Make a playable game of Lights Out. (Example web application with an unattractive interface.)