Lights Out
The game of Lights Out. (Example web application with an unattractive interface.)
The model should be a list of Point
, the locations that are
“lit up”.
type Model = [Point]
Requirement: you need to write enough of your own testing code (“check-expects”) to prove that each function works as advertised.
Draw Handler
Write a function to draw the board based on the list of Point
provided.
I chose to use the numbers [(-2)..2]
for each of my
Point
coordinates… so not the actual centers of the
circles. (Sometimes these are called “grid coordinates”.)
As part of this exercise you must not just use the raw coordinates for the center of each circle to represent it.
render :: Model -> Picture
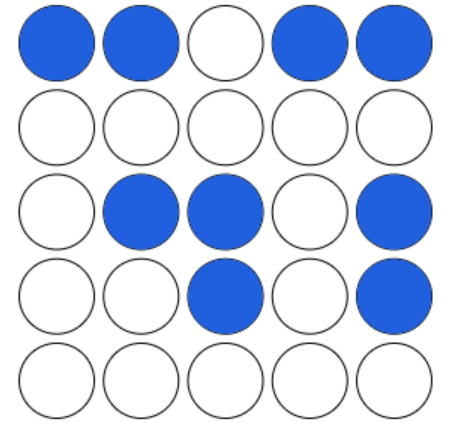
After everything else works, you should add a “win screen” – a victory message that shows when all of the lights are out.
Game Logic
-
clickedPt :: Point -> Maybe Point
Given the coordinates of a click, return the coordinates of the center of the circle that was clicked on. Give
Nothing
if no circle was clicked on. -
flipOne :: Model -> Point -> Model
Change the state of one circle in the model. (That is, flip between solid blue and outline.) If the given circle is in the list, take it out; otherwise, put it in.
-
flipAll :: Model -> [Point] -> Model
Change the state of all of the circles in the list (the second argument).
-
updateClick :: Model -> Maybe Point -> Model
Ignore
Nothing
clicks, otherwise flip the clicked circle and all of its neighbors (just like happens in the game).
Event Handler
Connecting the game play to the mouse action should be straightforward.
Finish by making an activityOf
your lights out game.
Playing
Warning: not all light configurations can be solved.
Observed Errors
Errors observed in student work.