Draw Handler
Assumed knowledge
If you have never drawn anything in CodeWorld, take half an hour and learn how to draw outline and solid shapes in various colors. You can read about Pictures in the Guide..
Minimum:
import CodeWorld
- circle
- solidCircle
- rectangle
- solidRectangle
- translated
- colored
- pictures
It is also helpful to know about coordinatePlane
so you understand
what numbers will appear as x and y coordinates of the mouse.
Step 0: warmup
Make a picture showing all of the following, together:
- a 3x2 solid blue rectangle centered at (4,5)
- a radius 3.5 circle centered at (-2,1)
- the coordinate plane
If you are “advanced”, add in a solid green triangle with vertices (-4,1), (0,-7), and (8,-5).
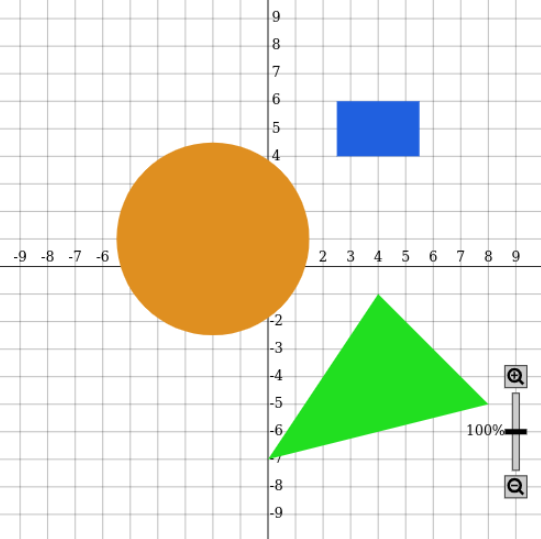
Step 1: background
The first step is to create the lines for the tic-tac-toe board. Draw a careful picture labeling coordinates and the width and height of everything, then the rest of the functions will be easier.
You should try this step yourself! As motivation, here is one possible board sketch:
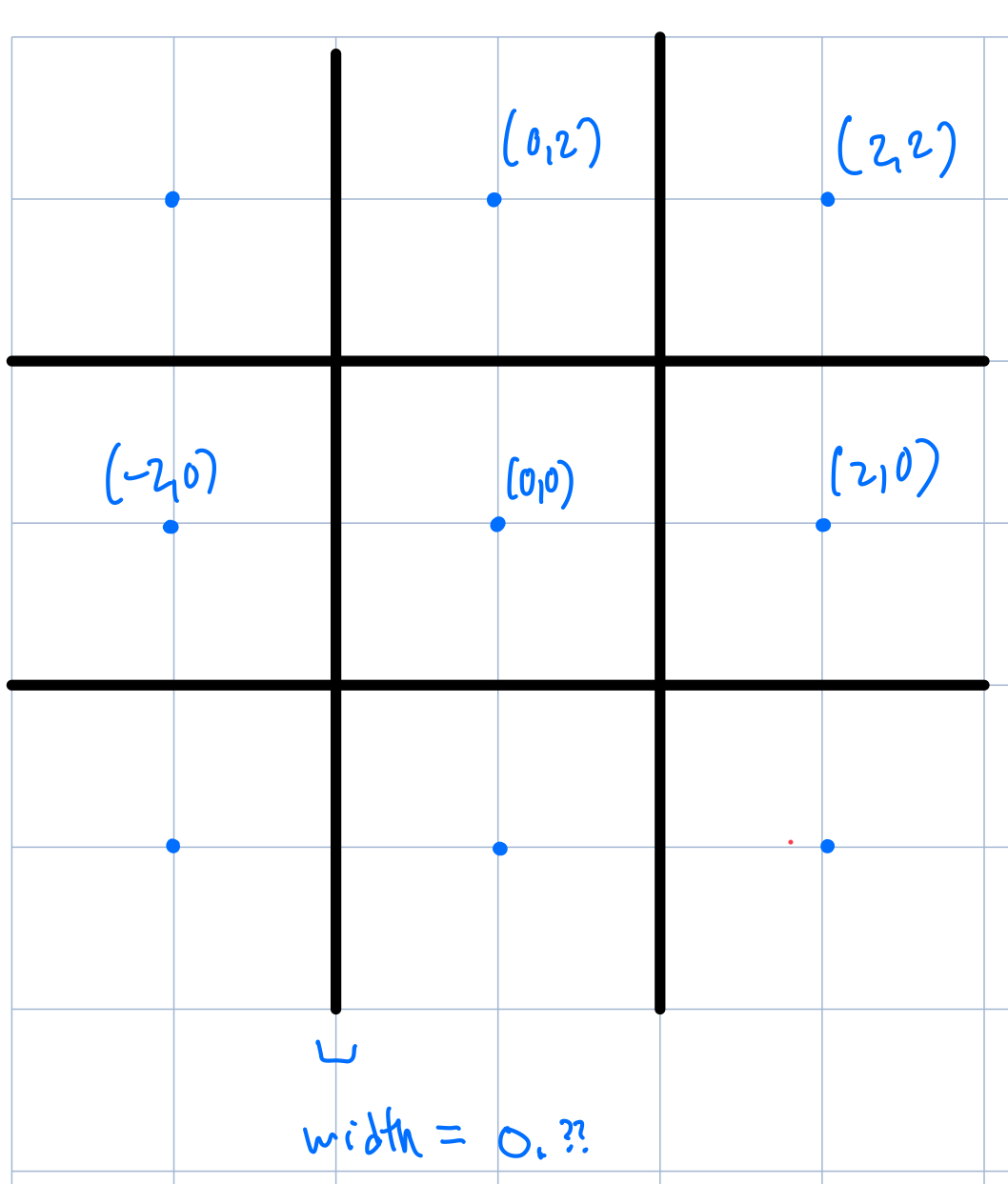
Step 2a: warmup for single piece
“Board coordinates” of (0,2) correspond to “computer coordinates” of (-2,2) using the board sketch above. Write a function whose input is the board coordinate of a square and whose output is the computer coordinate of that square (use your drawing from step 1).
Of course you could just write nine different statements like the one below, but a little bit of math makes it a lot less annoying.
compcoord :: Posn -> Posn
compcoord (0,2) == (-2,2)
compcoord (1,0) == (0,-2)
-- write a math function!
Step 2b: draw single piece
The second step is to write a function that renders a single piece.
drawPiece :: Piece -> Picture
The most common problem here is that the coordinates given in the
piece are integers like (0,2) but translated
wants decimals
(Double
). Use the fromIntegral
function.
Example: the demo function below shows how to draw a 50x50 blue square at coordinates (-3,1), (0,1) or (3,1) depending on what number comes in.
import CodeWorld
demo :: Int -> Picture
demo n = translated newx 1.0 $ colored blue $ solidRectangle 0.5 0.5
where newx = 3.0 * (fromIntegral n)
main = drawingOf $ pictures [demo 1, coordinatePlane]
Step 3: draw a list of pieces
The final step is to render a whole list of pieces. Don’t try to write this function until you are happy with your one piece drawing. After that it should be a small step.