2022 Review 2
- What is the difference between
for/list
andfor*/list
? Give an example and explain in a sentence.
A traditional checkerboard has alternating red and black squares. It is oriented so the red (“light”) square is on the near right from each player’s perspective. Pieces only go on the black (“dark”) squares. The squares I use are 50x50.
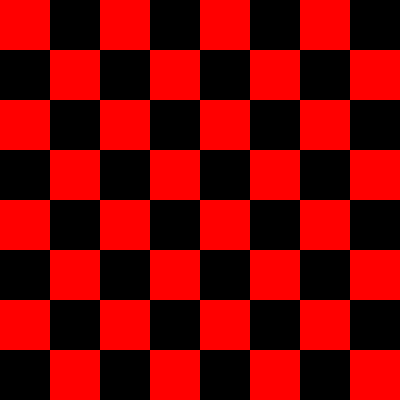
- Use
for/fold
to construct the checkerboard.
You will make a model using these structures:
;; Piece is a structure containing:
;; pos: a Posn, the board coordinates of the center of the piece
;; team: a number, 0 or 1
(define-struct piece (pos team))
;; M is a structure containing:
;; pieces: a list of Piece, the current state of the board
;; selected: a Posn, the square that is selected, or (-1,-1) for no selection
(define-struct m (pieces selected))
If they print out in a way that you cannot see what is inside, like
this: (make-m ...)
, add the #:prefab
tag you have been using for
messages.. You could use #:transparent
instead, it prints out a
little nicer.
-
Use
for/list
to create a list of all of the checkers. Here is an example of a black checker at (x=2, y=1):(make-piece (make-posn 2 1) 1)
. See the diagram below for checker positions. -
Write a draw handler that takes in an
m
and produces anImage
. As before, usefor/fold
. -
Write the
move-checker
function that takes in a list of pieces, a start posn, and an end posn. It should move all of the pieces located at the start posn to the end posn. Usefor/list
. -
(Coordinate conversions) Write functions that convert computer (x,y) coordinates to board (x,y) coordinates and vice-versa. Since my squares are 50x50, any mouse click with $0 \le x < 50$ and $0\le y< 50$ is considered $(0,0)$ on the board. Another example is that that $(35, 80)$ maps to board coordinates $(1,2)$. You can do this your own
invented way, but make sure clicking off-center still works the same as clicking in the exact center of a square.
(check-expect (c->b (make-posn 49 48)) (make-posn 0 0)) (check-expect (c->b (make-posn 35 80)) (make-posn 0 1)) (check-expect (b->c (make-posn 0 0)) (make-posn 25 25)) (check-expect (b->c (make-posn 1 2)) (make-posn 75 125))
-
(Mouse Handler) When the
selected
field is(make-posn -1 -1)
, the mouse handler sets theselected
field to be the center of the square clicked on. When theselected
field is not (-1, -1), then the mouse handler moves the piece usingmove-checker
.(check-expect (mouse-h (make-m (list (make-piece (make-posn 1 3) 1) (make-piece (make-posn 1 5) 0)) (make-posn -1 -1)) 80 210 "button-down") (make-m (list (make-piece (make-posn 1 3) 1) (make-piee (make-posn 1 5) 0)) (make-posn 1 4))) (check-expect (mouse-h (make-m (list (make-piece (make-posn 1 3) 1) (make-piece (make-posn 1 5) 0)) (make-posn 1 4)) 80 210 "button-down") (make-m (list (make-piece (make-posn 1 3) 1) (make-piee (make-posn 1 5) 0)) (make-posn 1 4)))
-
(Big Bang) Put together your animation and verify that you can move the pieces. You are not asked to follow any rules about what is a legal move.